I’m dabbling with Vue version 3. Here’s Part 2 of 2 in generating a reusable component. This time with an API call attached.
Introduction
Let’s build this. A simple Vue app which accesses Github’s REST API and prints a user’s profile into a Bootstrap 4 card interface.
What’s the Goal?
Create a reusable component that displays a person’s Github profile.
What will we need?
- Stackblitz – to quickly prototype a Vue project and its dependencies.
- Bootstrap 4 – to grab some ready-made user interfaces (e.g. cards).
- Axios – the promise based library we’ll use to communicate with Github’s API.
- Github API – the data source we’ll need to access and display a user’s profile.
Step By Step
Step 1: Create Vue App
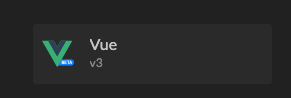
Step 2: Install Dependencies

Step 3: Add Dependencies to main.js
const { createApp } = require("vue");
import App from "./App.vue";
import "bootstrap"; // Add this import.
import "bootstrap/dist/css/bootstrap.css"; // Add this import.
createApp(App).mount("#app");
Step 4: Create a Service for API Call

GithubAPI.js.
The Service Code
Debrief of the GithubAPI service code:
- Line 1: Import
axios
library for promise based HTTP requests. - Line 5: Write a function called
getGithubProfile()
and pass inusername
as an argument. - Line 7: Leverage the get method and concatenate the
username
variable. - Line 9: Return the
response
object (component will consume this).
Step 5: Modify HelloWorld.vue Component
Out of box, Stackblitz generates a default Vue project paired with a component called HelloWorld.vue.
Rename it.

GithubProfile.vue.
The Component Code
Debrief of the GithubProfile component code:
- Lines 3-16: The Bootstrap card.
- Line 20: Import the service made from Step 4.
- Lines 23-28: The prop you’ll pass in (e.g. a profile name).
- Lines 29-33: Setup Vue’s data object which will populate the card.
- Lines 34-42: Setup the API call inside of the created lifecycle hook.
Debrief of the App component code:
- Line 13: the prop passed in as a string (e.g. my profile name).
Key Takeaways
We’ve now created a reusable component which is dynamic enough to apply a Bootstrap card by passing in the Github profile as a prop. This will use Github’s API to make a call on their end which will print the profile data to the card.
Additional Resources
- Vue School’s free component course.
- Source code for this demo is available on Github and Stackblitz.
- If you want to make a context switch to React, a similar implementation can be found here.